An error occurred while processing your request.
Request ID: 00-a320a207cd79a79af1b500140bae4593-b6b18f0ebc41c41f-00
Error Code: 404 - Page Not Found
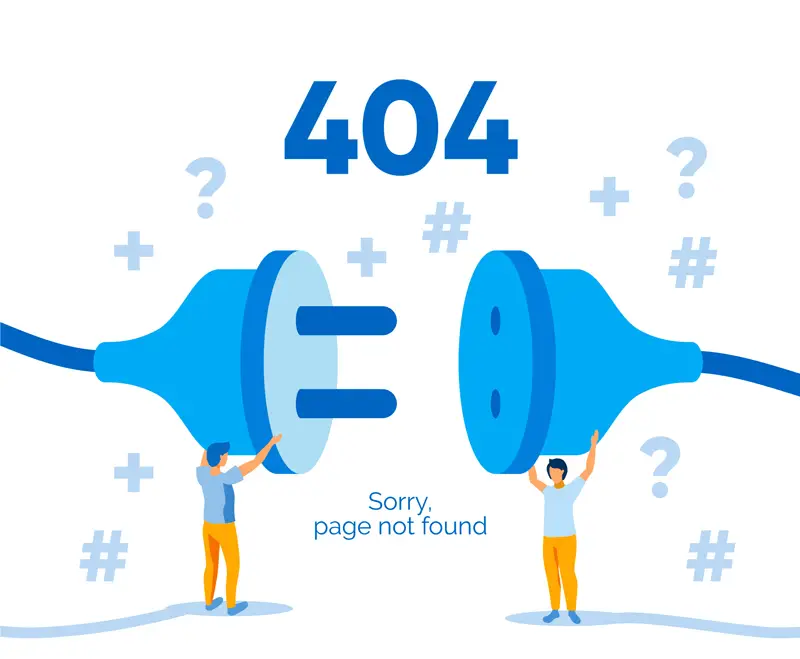
Categories
Popular Posts
Structured Data using FoxLearn.JsonLd
Jun 20, 2025
Implement security headers for an ASP.NET Core
Jun 24, 2025
10 Common Mistakes ASP.NET Developers Should Avoid
Dec 16, 2024
Modular Admin Template
Nov 14, 2024
SB Admin Template
Nov 14, 2024